原生ajax、axios、fetch配合php上传文件教程
日期:2018-08-26
来源:程序思维浏览:1791次
最近在用ajax、fetch、axios配合php做文件上传功能,在网上搜索好多文章,都有一些小问题,我把整理好的代码教程写出来供大家学习。
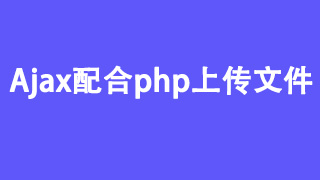
先看服务端php里面的代码:
upload.php里面的代码:
<?php
require("UploadFile.class.php");
$uf=new UploadFile();
$uf->upfileload("fileName",'./uploadfiles',array("jpg","gif","png","jpeg"),1024);
UploadFile.class.php里面的代码:
<?php
set_time_limit(0);
class UploadFile{
protected $filepath; //上传路径
protected $fileinfo; //文件信息
protected $extfile; //文件扩展名
protected $filename; //文件名
protected $filesizes; //文件大小
protected $UpFileType;//上传文件类型
protected $myfile;//formfiles.js里的文件上传路径
public $msg;
//执行上传文件
function upfileload($postname,$filepath,$upfiletype,$setsize,$action='',$refile=''){
$this->fileoption($postname,$upfiletype,$filepath);
if($this->fileError($this->extfile,$this->UpFileType)){
$this->delReFile($refile);
$this->upfiles($this->filesizes,$this->filepath,$this->filename,$setsize);
}
}
//删除修改时已上传的文件
private function delReFile($refile){
if(is_file($refile)){
@unlink($refile);
}
}
//获取文件属性
private function fileoption($postname,$FileType,$filepath){
$this->myfile=$postname;
$this->fileinfo=pathinfo($_FILES["{$this->myfile}"]["name"]); //获取文件信息
$this->extfile=strtolower($this->fileinfo["extension"]); //获取文件扩展名
$this->filename=$this->uniqueId().".".$this->extfile; //自动生成文件名
$this->filesizes=$_FILES["{$this->myfile}"]["size"]/1024;//1kB
$this->UpFileType=$FileType;//获取文件类型:设置模式array("jpg","gif","png","jpeg")
$this->filepath=$filepath."/".$this->filename;//获取文件路径
}
//设置文件错误提示
private function fileError($extfile,$upfiletype){
if ($_FILES["{$this->myfile}"]["error"]>0){
switch($_FILES["{$this->myfile}"]["error"]){
case 1:
$this->msg="{\"msg\":\"0\",\"msbox\":\"上传文件超过了php.ini中upload_max_filesize这个选项设置的值\"}";
echo $this->msg;
return false;
break;
case 2:
$this->msg="{\"msg\":\"0\",\"msbox\":\"上传的文件大小超过了HTML表单中MAX_FILE_SIZE选项指定的值\"}";
echo $this->msg;
return false;
break;
case 3:
$this->msg="{\"msg\":\"0\",\"msbox\":\"文件只有部分被上传\"}";
echo $this->msg;
return false;
break;
case 4:
$this->msg="{\"msg\":\"0\",\"msbox\":\"没有文件上传\"}";
echo $this->msg;
return false;
break;
}
}else{
if (!in_array($extfile,$upfiletype) || preg_match('/(<\?php)|(<script)|(<html)|(<iframe)|(<body)/i',file_get_contents($_FILES["{$this->myfile}"]["tmp_name"]),$con)){
$this->msg="{\"msg\":\"0\",\"msbox\":\"文件类型上传不正确\"}";
echo $this->msg;
return false;
}else{
return true;
}
}
}
//执行文件上传
private function upfiles($filesize,$filepath,$filename,$setsize){
if(is_uploaded_file($_FILES["{$this->myfile}"]["tmp_name"])){
if($filesize<$setsize){
if(move_uploaded_file($_FILES["{$this->myfile}"]["tmp_name"],$filepath)){
$this->msg="{\"msg\":\"1\",\"msbox\":\"".$filename."\"}";
}else{
$this->msg="{\"msg\":\"0\",\"msbox\":\"上传文件失败\"}";
}
}else{
$this->msg="{\"msg\":\"0\",\"msbox\":\"文件大小超过了限制\"}";
}
echo $this->msg;
}
}
private function uniqueId(){
$qidarr=@explode(".",uniqid('',true));
$qid=rand(1,9).$qidarr[1];
return $qid;
}
}
?>
1、原生js里面的ajax文件上传
upload.html里面的代码:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>ajax文件上传</title>
</head>
<body>
<form action="" method="post" enctype="multipart/form-data">
<input type="file" id="imgfile" onchange="uploadImageFile()" />
</form>
<script>
function uploadImageFile(){
var xhr = new XMLHttpRequest();
//定义表单变量
var file = document.getElementById('imgfile').files[0];
//新建一个FormData对象
var formData = new FormData();
formData.append("fileName",file);
//监听上传进度
xhr.upload.onprogress=function(e){
//console.log(e);
var percentCompleted = Math.round( (e.loaded * 100) / e.total );
console.log(percentCompleted);
}
//post方式
xhr.open('POST', "upload.php");
//发送请求
xhr.send(formData);
//success回调
xhr.onreadystatechange = function(){
if ( xhr.readyState == 4 && xhr.status == 200 ) {
//console.log( xhr.responseText );
//var data = xhr.responseText;
var data = JSON.parse(xhr.responseText)
console.log(data)
}
};
//设置超时时间
xhr.timeout = 100000;
xhr.ontimeout = function(event){}
}
</script>
</body>
</html>
原生ajax文件上传教程完毕。
2、axios文件上传
upload.html里面的代码:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>axios文件上传</title>
</head>
<body>
<form action="" method="post" enctype="multipart/form-data">
<input type="file" id="imgfile" onchange="uploadImageFile()" />
</form>
<script src="https://cdn.bootcss.com/axios/0.18.0/axios.min.js"></script>
<script>
function uploadImageFile(){
//定义表单变量
var file = document.getElementById('imgfile').files[0];
//新建一个FormData对象
var formData = new FormData();
formData.append("fileName",file);
var config = {
//监听上传进度
onUploadProgress: function(progressEvent) {
var percentCompleted = Math.round( (progressEvent.loaded * 100) / progressEvent.total );
console.log(percentCompleted);
}
};
axios.post('upload.php', formData, config)
.then(function (res) {
console.log(res);
})
.catch(function (err) {
console.log(err);
});
}
</script>
</body>
</html>
axios文件上传教程完毕。
3、fetch文件上传
upload.html里面的代码:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>fetch文件上传</title>
</head>
<body>
<form action="" method="post" enctype="multipart/form-data">
<input type="file" id="imgfile" onchange="uploadImageFile()" />
</form>
<script>
function uploadImageFile(){
//定义表单变量
var file = document.getElementById('imgfile').files[0];
//新建一个FormData对象
var formData = new FormData();
//追加文件数据
formData.append("fileName",file);
fetch('upload.php', {
method: 'POST',
body: formData
}).then((res)=>{
return res.json();
}).then(res=>{
console.log(res)
});
}
</script>
</body>
</html>
fetch文件上传教程完毕。
源码下载
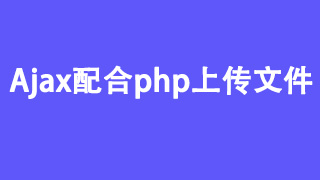
先看服务端php里面的代码:
upload.php里面的代码:
<?php
require("UploadFile.class.php");
$uf=new UploadFile();
$uf->upfileload("fileName",'./uploadfiles',array("jpg","gif","png","jpeg"),1024);
UploadFile.class.php里面的代码:
<?php
set_time_limit(0);
class UploadFile{
protected $filepath; //上传路径
protected $fileinfo; //文件信息
protected $extfile; //文件扩展名
protected $filename; //文件名
protected $filesizes; //文件大小
protected $UpFileType;//上传文件类型
protected $myfile;//formfiles.js里的文件上传路径
public $msg;
//执行上传文件
function upfileload($postname,$filepath,$upfiletype,$setsize,$action='',$refile=''){
$this->fileoption($postname,$upfiletype,$filepath);
if($this->fileError($this->extfile,$this->UpFileType)){
$this->delReFile($refile);
$this->upfiles($this->filesizes,$this->filepath,$this->filename,$setsize);
}
}
//删除修改时已上传的文件
private function delReFile($refile){
if(is_file($refile)){
@unlink($refile);
}
}
//获取文件属性
private function fileoption($postname,$FileType,$filepath){
$this->myfile=$postname;
$this->fileinfo=pathinfo($_FILES["{$this->myfile}"]["name"]); //获取文件信息
$this->extfile=strtolower($this->fileinfo["extension"]); //获取文件扩展名
$this->filename=$this->uniqueId().".".$this->extfile; //自动生成文件名
$this->filesizes=$_FILES["{$this->myfile}"]["size"]/1024;//1kB
$this->UpFileType=$FileType;//获取文件类型:设置模式array("jpg","gif","png","jpeg")
$this->filepath=$filepath."/".$this->filename;//获取文件路径
}
//设置文件错误提示
private function fileError($extfile,$upfiletype){
if ($_FILES["{$this->myfile}"]["error"]>0){
switch($_FILES["{$this->myfile}"]["error"]){
case 1:
$this->msg="{\"msg\":\"0\",\"msbox\":\"上传文件超过了php.ini中upload_max_filesize这个选项设置的值\"}";
echo $this->msg;
return false;
break;
case 2:
$this->msg="{\"msg\":\"0\",\"msbox\":\"上传的文件大小超过了HTML表单中MAX_FILE_SIZE选项指定的值\"}";
echo $this->msg;
return false;
break;
case 3:
$this->msg="{\"msg\":\"0\",\"msbox\":\"文件只有部分被上传\"}";
echo $this->msg;
return false;
break;
case 4:
$this->msg="{\"msg\":\"0\",\"msbox\":\"没有文件上传\"}";
echo $this->msg;
return false;
break;
}
}else{
if (!in_array($extfile,$upfiletype) || preg_match('/(<\?php)|(<script)|(<html)|(<iframe)|(<body)/i',file_get_contents($_FILES["{$this->myfile}"]["tmp_name"]),$con)){
$this->msg="{\"msg\":\"0\",\"msbox\":\"文件类型上传不正确\"}";
echo $this->msg;
return false;
}else{
return true;
}
}
}
//执行文件上传
private function upfiles($filesize,$filepath,$filename,$setsize){
if(is_uploaded_file($_FILES["{$this->myfile}"]["tmp_name"])){
if($filesize<$setsize){
if(move_uploaded_file($_FILES["{$this->myfile}"]["tmp_name"],$filepath)){
$this->msg="{\"msg\":\"1\",\"msbox\":\"".$filename."\"}";
}else{
$this->msg="{\"msg\":\"0\",\"msbox\":\"上传文件失败\"}";
}
}else{
$this->msg="{\"msg\":\"0\",\"msbox\":\"文件大小超过了限制\"}";
}
echo $this->msg;
}
}
private function uniqueId(){
$qidarr=@explode(".",uniqid('',true));
$qid=rand(1,9).$qidarr[1];
return $qid;
}
}
?>
1、原生js里面的ajax文件上传
upload.html里面的代码:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>ajax文件上传</title>
</head>
<body>
<form action="" method="post" enctype="multipart/form-data">
<input type="file" id="imgfile" onchange="uploadImageFile()" />
</form>
<script>
function uploadImageFile(){
var xhr = new XMLHttpRequest();
//定义表单变量
var file = document.getElementById('imgfile').files[0];
//新建一个FormData对象
var formData = new FormData();
formData.append("fileName",file);
//监听上传进度
xhr.upload.onprogress=function(e){
//console.log(e);
var percentCompleted = Math.round( (e.loaded * 100) / e.total );
console.log(percentCompleted);
}
//post方式
xhr.open('POST', "upload.php");
//发送请求
xhr.send(formData);
//success回调
xhr.onreadystatechange = function(){
if ( xhr.readyState == 4 && xhr.status == 200 ) {
//console.log( xhr.responseText );
//var data = xhr.responseText;
var data = JSON.parse(xhr.responseText)
console.log(data)
}
};
//设置超时时间
xhr.timeout = 100000;
xhr.ontimeout = function(event){}
}
</script>
</body>
</html>
原生ajax文件上传教程完毕。
2、axios文件上传
upload.html里面的代码:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>axios文件上传</title>
</head>
<body>
<form action="" method="post" enctype="multipart/form-data">
<input type="file" id="imgfile" onchange="uploadImageFile()" />
</form>
<script src="https://cdn.bootcss.com/axios/0.18.0/axios.min.js"></script>
<script>
function uploadImageFile(){
//定义表单变量
var file = document.getElementById('imgfile').files[0];
//新建一个FormData对象
var formData = new FormData();
formData.append("fileName",file);
var config = {
//监听上传进度
onUploadProgress: function(progressEvent) {
var percentCompleted = Math.round( (progressEvent.loaded * 100) / progressEvent.total );
console.log(percentCompleted);
}
};
axios.post('upload.php', formData, config)
.then(function (res) {
console.log(res);
})
.catch(function (err) {
console.log(err);
});
}
</script>
</body>
</html>
axios文件上传教程完毕。
3、fetch文件上传
upload.html里面的代码:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>fetch文件上传</title>
</head>
<body>
<form action="" method="post" enctype="multipart/form-data">
<input type="file" id="imgfile" onchange="uploadImageFile()" />
</form>
<script>
function uploadImageFile(){
//定义表单变量
var file = document.getElementById('imgfile').files[0];
//新建一个FormData对象
var formData = new FormData();
//追加文件数据
formData.append("fileName",file);
fetch('upload.php', {
method: 'POST',
body: formData
}).then((res)=>{
return res.json();
}).then(res=>{
console.log(res)
});
}
</script>
</body>
</html>
fetch文件上传教程完毕。
源码下载
精品好课